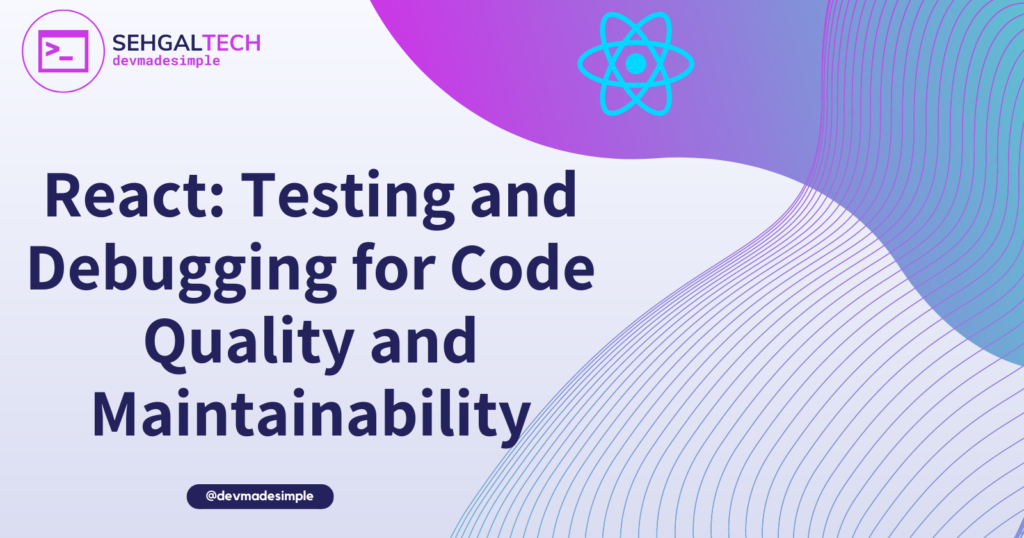
Welcome back to the React 101 series! In our journey through React development, we’ve covered components, state management, and styling. Now, it’s time to focus on two crucial aspects of software development: testing and debugging. These practices are essential for ensuring code quality, maintainability, and a smooth user experience.. In this post, we’ll explore the importance of testing, different testing approaches, and best practices for debugging your React applications.
Why is Testing Important in React?
Testing is an integral part of the development process that helps identify and fix issues early on. It contributes to code quality, reduces bugs, and enhances maintainability.
Testing plays a vital role in:
- Catching bugs early: Tests help identify issues early in the development process, preventing them from reaching production and impacting users.
- Improving code quality: Writing tests forces you to think about the behavior of your components and potential edge cases, leading to cleaner and more maintainable code.
- Confidence in Changes: Tests provide a safety net when making code changes, giving you confidence that existing functionalities remain intact.
React applications can be tested at various levels, including unit testing, integration testing, and end-to-end testing.
1. Unit Testing:
Unit testing involves testing individual units or components of your application in isolation. In React, tools like Jest and testing-library/react are commonly used for unit testing. Writing tests for components ensures that they behave as expected and helps catch regressions.
// Example: Jest unit test
import { render, screen } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders hello message', () => {
render(<MyComponent />);
expect(screen.getByText('Hello, React!')).toBeInTheDocument();
});
2. Integration Testing:
These tests verify how multiple components interact with each other and with external APIs or services. Tools like Jest and Enzyme are commonly used for integration testing in React. This type of testing ensures that the components work together as intended.
// Example: Jest + Enzyme integration test
import { shallow } from 'enzyme';
import MyComponent from './MyComponent';
test('renders without errors', () => {
const wrapper = shallow(<MyComponent />);
expect(wrapper.exists()).toBe(true);
});
3. End-to-End Testing:
End-to-End (E2E) testing involves testing the entire application from start to finish. Tools like Cypress and Puppeteer are commonly used for E2E testing. These tests simulate user interactions and verify that the application behaves correctly.
// Example: Cypress end-to-end test
describe('MyComponent', () => {
it('renders hello message', () => {
cy.visit('/');
cy.contains('Hello, React!').should('be.visible');
});
});
Best Practices for Testing in React
- Write Testable Code: Design your components with testability in mind. Keep your components small and focused on a single responsibility, making it easier to write meaningful tests.
- Use Test Frameworks: Leverage popular testing frameworks like Jest for unit testing and testing-library/react for testing React components. These frameworks provide powerful tools and utilities for writing effective tests.
- Mock External Dependencies: When testing components that interact with external services or APIs, use mocks to isolate the component and avoid real network requests. This ensures consistent and controlled test environments.
- Continuous Integration (CI): Integrate testing into your CI/CD pipeline to automatically run tests whenever code changes are pushed. This helps catch issues early and ensures that new code doesn’t break existing functionality.
- Snapshot Testing: Use snapshot testing to capture the expected output of a component and compare it with future renders. This can be especially useful for quickly identifying unintentional changes.
Effective Debugging Strategies
Debugging is a crucial skill for developers, and React provides several tools and techniques to make the process more efficient.
1. Use React DevTools: React DevTools is a browser extension that allows you to inspect the React component hierarchy, view component props and state, and even modify them in real-time. It’s a powerful tool for debugging React applications.
2. Console Logging: Simple yet effective, adding console logs to your code can help you trace the flow of data and identify the source of issues. Use console.log
strategically to output relevant information.
const MyComponent = () => {
console.log('Rendering MyComponent');
// ... rest of the component code
};
3. Debugging Tools in IDEs: Modern integrated development environments (IDEs) like Visual Studio Code provide powerful debugging tools. Set breakpoints, inspect variables, and step through your code to identify and fix issues.
4. React Error Boundaries: Implement error boundaries to catch JavaScript errors anywhere in a component tree. This prevents the entire application from crashing and allows you to gracefully handle errors.
class ErrorBoundary extends React.Component {
componentDidCatch(error, errorInfo) {
logErrorToService(error, errorInfo);
}
render() {
return this.props.children;
}
}
5. React Strict Mode: Enable React Strict Mode in development to catch common mistakes, such as side effects during rendering. It provides additional warnings and helps you write safer code.
import React from 'react';
const App = () => {
return (
<React.StrictMode>
{/* Your app components */}
</React.StrictMode>
);
};
Conclusion
Testing and debugging are integral components of the software development lifecycle, ensuring that your React applications are robust, reliable, and maintainable. By adopting testing best practices, using effective debugging strategies, and leveraging the tools provided by the React ecosystem, you can build high-quality applications that meet user expectations. In the next part of the React 101 series, we’ll explore more advanced topics like performance optimisation techniques, so stay tuned for more React insights!