JavaScript is a versatile programming language used for creating interactive websites and web applications. It is a dynamically typed language, which means variables can hold different data types at different times. Understanding the concept of variable scope is essential to write efficient and reliable JavaScript code.
In JavaScript, there are three different ways to declare variables: let
, var
, and const
. Each of these keywords has its own unique characteristics and usage. In this blog post, we will discuss the differences between let
vs var
vs const
, and when to use each of them.
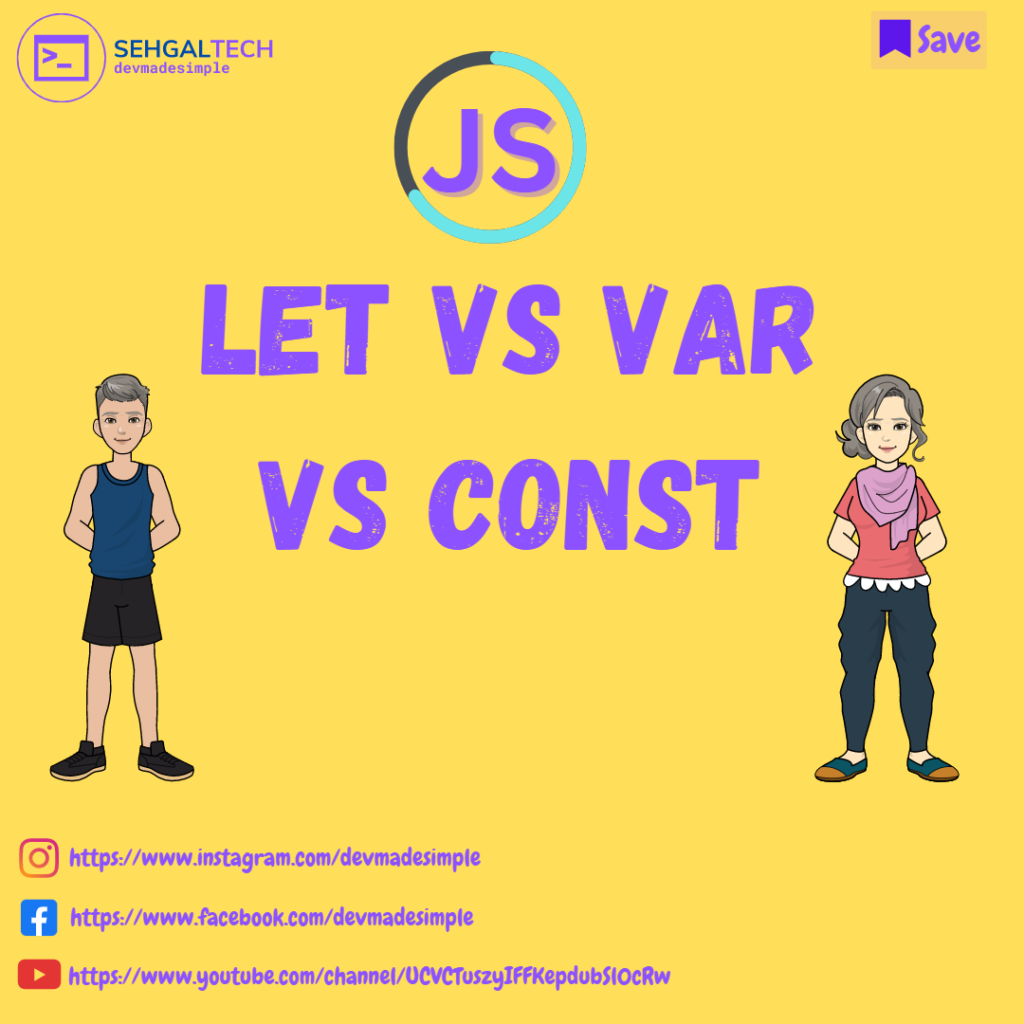
What is variable scope?
Variable scope refers to the accessibility of variables in a program. In other words, it determines where a variable can be accessed and modified. Every programming language has its own set of rules for variable scope. In JavaScript, the scope of a variable is determined by where it is declared.
Types of variable scope in JavaScript
JavaScript has three types of variable scope:
Global Scope
Variables declared outside of any function or block are called global variables. These variables are accessible throughout the program, including within functions and blocks.
var name = "John"; // Global variable
function greet() {
console.log("Hello, " + name + "!");
}
greet(); // Output: Hello, John!
In the above example, the name
variable is declared outside of the greet
function, making it a global variable. The greet
function can access the name
variable and print it to the console.
However, using global variables can be risky as they can be accidentally modified by any part of the program. It can lead to unexpected results and bugs, making the code difficult to debug and maintain.
Local Scope
Variables declared inside a function or block are called local variables. These variables are accessible only within the function or block where they are declared.
function greet() {
var name = "John"; // Local variable
console.log("Hello, " + name + "!");
}
greet(); // Output: Hello, John!
In the above example, the name
variable is declared inside the greet
function, making it a local variable. The name
variable is only accessible within the greet
function.
Local variables have limited scope, making it easier to maintain and debug the code. It also prevents accidental modifications from other parts of the program.
Block scope
Variables declared inside a block, such as a for
loop or an if
statement, are considered to have block scope. Block-scoped variables can only be accessed and modified within the block in which they are defined, including any nested blocks.
function foo() {
if (true) {
let blockVar = "I am a block-scoped variable";
console.log(blockVar);
}
console.log(blockVar); // Output: ReferenceError: blockVar is not defined
}
foo();
In the above example, the blockVar variable is declared inside an if block, so it has block scope. The console.log() statement inside the block can access and log the value of blockVar, but the console.log() statement outside the block generates an error because blockVar is not defined in the outer scope.
Variable scope chain
In JavaScript, the scope chain is the hierarchy of variable scope that the JavaScript engine follows to find the value of a variable. When a variable is accessed, the JavaScript engine first searches for the variable within the local scope. If it is not found, it searches in the next outer scope until it reaches the global scope.
var name = "John"; // Global variable
function greet() {
var message = "Hello, " + name + "!"; // Accessing global variable
console.log(message);
}
greet(); // Output: Hello, John!
In the above example, the message variable is declared inside the greet function, making it a local variable. However, the name variable is not declared inside the greet function, making it a global variable. The greet function can access the name variable because it is in the global scope.
Scope of let
The let
keyword was introduced in ES6 (ECMAScript 2015) for declaring block-scoped variables. A block is defined as any code between curly braces {}
. Variables declared with let
have block scope, which means they are only accessible within the block they are defined in, including nested blocks.
let x = 1;
if (true) {
let x = 2;
console.log(x); // Output: 2
}
console.log(x); // Output: 1
In the above example, the variable x
is declared twice. The first declaration is outside the if block, and the second declaration is inside the if block. Each declaration of x
creates a new variable with a different scope. The variable x
inside the if block is different from the variable x
outside the if block because it has block scope.
Scope of var
The var
keyword has been used in JavaScript since its inception for declaring variables. Variables declared with var
have function scope or global scope, which means that they are accessible throughout the function or program.
var x = 1;
if (true) {
var x = 2;
console.log(x); // Output: 2
}
console.log(x); // Output: 2
In the above example, the variable x
is declared twice, just like in the previous example. However, because var
has function scope, both declarations of x
refer to the same variable. The value of x
is changed inside the if block and remains changed outside the if block because it has global scope.
Scope of const
The const
keyword was also introduced in ES6 for declaring constants. Variables declared with const
have block scope, just like let
. However, variables declared with const
cannot be reassigned or re-declared within the same scope.
const x = 1;
if (true) {
const x = 2;
console.log(x); // Output: 2
}
console.log(x); // Output: 1
In the above example, the variable x
is declared twice, just like in the previous examples. However, because const
variables cannot be reassigned or re-declared within the same scope, each declaration of x
creates a new constant with a different scope. The variable x
inside the if block is different from the variable x
outside the if block because it has block scope and cannot be reassigned.
Using variable scope effectively
Understanding variable scope is essential to write efficient and reliable JavaScript code. Here are some tips to use variable scope effectively:
- Use local variables whenever possible to avoid accidental modifications and improve performance.
- Avoid using global variables unless necessary, as they can lead to unexpected results and bugs.
- Use variable names that are descriptive and meaningful to improve code readability and maintainability.
- Use block scope with
let
andconst
keywords instead ofvar
to declare variables within blocks.
Conclusion
Understanding variable scope is crucial to writing efficient and bug-free JavaScript code. Here are some key takeaways:
- Use
let
for variables that need to be reassigned within the same block. - Use
var
sparingly, if at all, as it can lead to unexpected results and bugs. - Use
const
for variables that should not be reassigned within the same block. This provides better security and reliability in the code. - Always declare variables with the correct scope to avoid unexpected results and bugs in your code.
By using let
, var
, and const
effectively, you can write clean, reliable, and efficient JavaScript code.