Welcome back to the React 101 series! In this chapter, we’ll explore two fundamental concepts that govern data flow and interaction in React components: props and state management. As building blocks of React, these concepts are crucial for creating dynamic and interactive user interfaces. Buckle up, and get ready to unlock the magic of building dynamic and interactive user interfaces!
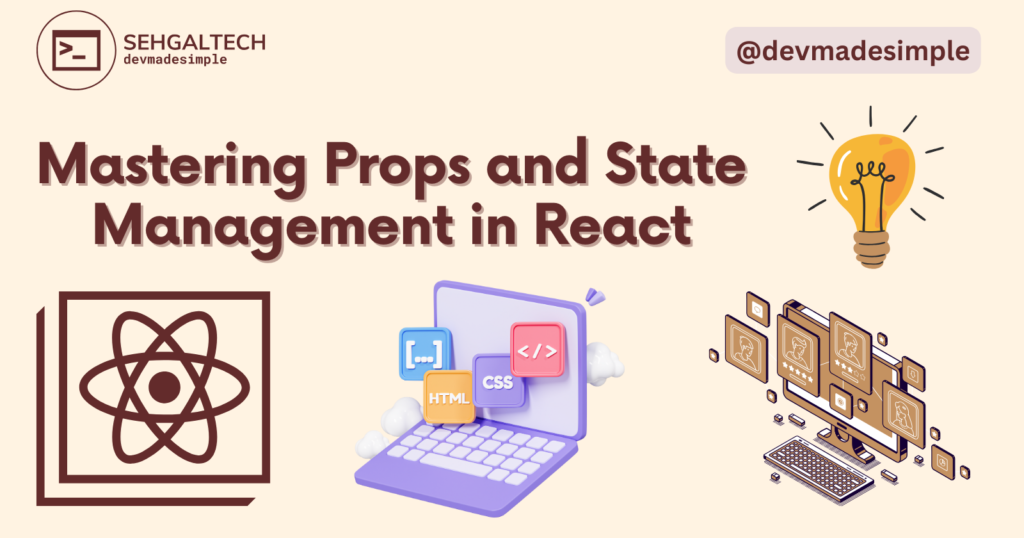
Props: Passing Data Between Components
Props, short for properties, facilitate the flow of data from parent to child components. Think of them as parameters passed to a function. In React, components act like functions, and props enable you to customize their behavior. Let’s explore the basics:
1. Passing Data Down the Component Tree:
// Parent Component
const App = () => {
const dataToPass = "Hello from Parent!";
return <ChildComponent message={dataToPass} />;
};
// Child Component
const ChildComponent = (props) => {
return <p>{props.message}</p>;
};
Here, the message
prop is passed from the parent (App
) to the child (ChildComponent
).
2. Using Props for Reusability:
Leverage props to create reusable components. By passing different data to the same component, you can reuse it across your application.
State: Managing Component Interactivity
State is a mechanism for a component to maintain and manage its own data. Unlike props, which are passed from parent to child, state is internal to the component.
1. Initializing State:
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
The useState
hook is used to declare state variables. In this example, count
is initialized to 0
, and the setCount
function updates its value.
2. Handling User Interactivity:
Components often need to respond to user actions. By using state, you can manage interactivity seamlessly.
const ToggleButton = () => {
const [isOn, setIsOn] = useState(false);
return (
<button onClick={() => setIsOn(!isOn)}>
{isOn ? "Turn Off" : "Turn On"}
</button>
);
};
Here, clicking the button toggles the isOn
state between true
and false
.
Bringing It Together: Props and State in Harmony
Combine props and state for powerful React components. Pass data via props to customize child components, and manage interactivity through state. Here’s a concise example:
const ParentComponent = () => {
const [message, setMessage] = useState("Hello from Parent!");
return (
<div>
<ChildComponent message={message} />
<button onClick={() => setMessage("Updated Message!")}>
Update Message
</button>
</div>
);
};
const ChildComponent = (props) => {
return <p>{props.message}</p>;
};
In this example, the ParentComponent
manages the message state and passes it to the ChildComponent
. Clicking the button updates the message in the parent, triggering a re-render.
Choosing the Right Tool for the Job
- Props: Use props for passing simple, static data down the component tree.
- State: Use state for managing internal data that changes within a component.
Beyond the Basics:
- Lifting state up: When state needs to be shared across multiple components, consider techniques like lifting state up to a common ancestor.
- State management libraries: For complex applications, explore state management libraries like Redux or MobX for centralized state management.
Remember the Key Points:
- Props and state are the foundation for data flow and interactivity in React.
- Props are one-way data flow from parent to child.
- State manages internal data within a component.
- Choose the right tool based on your data needs and complexity.
Conclusion:
Understanding the dynamics of props and state management is foundational to mastering React. As you explore more complex applications, these concepts will be your allies in creating modular, reusable, and interactive components. Stay tuned for the next installment in the React 101 Learning Series! Happy coding!