Text is a fundamental element in any mobile application, as it conveys information, instructions, and messages to users. In React Native, rendering text is achieved through the Text
component, which provides a simple yet powerful way to display text content in your app. In this blog, we’ll dive into the React Native Text Component, explore its features, and learn how to leverage its capabilities to create beautiful and responsive text displays in your React Native apps.
The Role of Text in Mobile Apps
Text plays a crucial role in mobile apps, serving various purposes:
- Content Display: Text is the primary means of presenting content to users, including headings, paragraphs, lists, and more.
- User Interaction: Text is used for buttons, links, and other interactive elements that allow users to navigate and perform actions.
- Accessibility: Providing text descriptions and labels is essential for making your app accessible to all users, including those with visual impairments.
Introducing the React Native Text Component
The React Native Text Component is a part of the React Native core library and comes pre-installed with any new React Native project. It is designed to render text and supports a wide range of features to customize its appearance.
Basic Usage
Using the React Native Text Component is straightforward. Here’s a basic example of how to render a simple text element:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const MyComponent = () => {
return (
<View style={styles.container}>
<Text>Hello, World!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
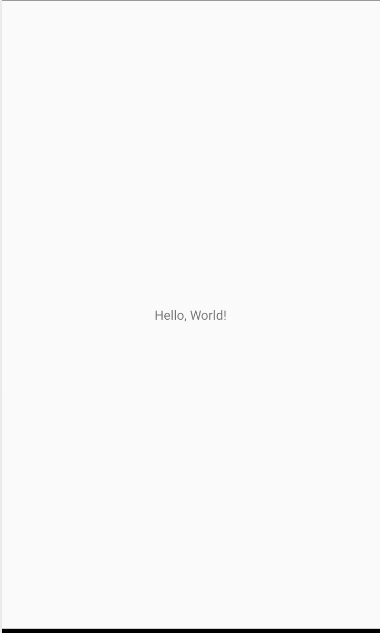
In this example, we import the necessary components from react-native
, and within the MyComponent
, we render the Text
component with the content “Hello, World!” inside a View
.
Customizing Text
The React Native Text Component provides several props that allow you to customize the appearance of text:
- style: The
style
prop allows you to apply various styles to the text, such as font size, color, weight, and more. You can use theStyleSheet.create
function to define a style object and apply it to theText
component. - numberOfLines: Use this prop to limit the number of lines the text occupies. This is particularly useful when dealing with long text strings to avoid overflowing the layout.
- textAlign: The
textAlign
prop enables you to align the text horizontally. Options include ‘left’, ‘center’, ‘right’, and ‘justify’. - lineHeight: This prop sets the height of each line of text, allowing you to control the spacing between lines.
Handling Text Content
Sometimes, text content may be dynamic or come from an external source like an API. To handle dynamic text content, you can interpolate JavaScript expressions within the Text
component using curly braces ‘{}’. Here’s an example:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const MyComponent = () => {
const greeting = 'Hello';
const name = 'John';
return (
<View style={styles.container}>
<Text>{`${greeting}, ${name}!`}</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
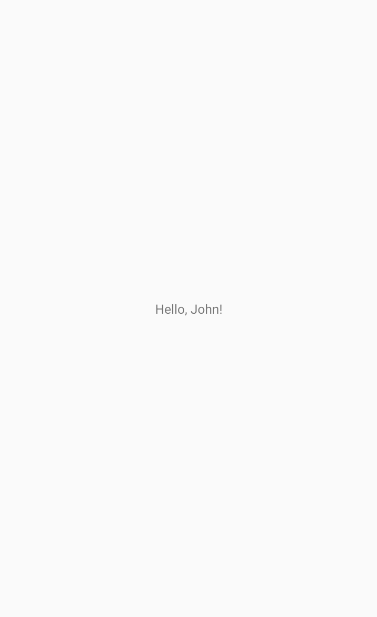
In this example, we’ve used template literals to display the greeting message “Hello, John!” dynamically.
Conclusion
The React Native Text Component is a powerful tool for rendering text in your mobile apps. Its flexibility and customization options allow you to create visually appealing and interactive text displays to enhance the user experience. Whether you’re displaying static content or handling dynamic data, the Text
component has got you covered.
As you continue to explore the world of React Native development, remember that text plays a pivotal role in communicating with users effectively. Utilize the React Native Text Component wisely, and your app’s text will be engaging and user-friendly.
Happy coding!