What is Linked List?
Imagine you have a chain of interconnected boxes. Each box has two parts: one part contains some data, and the other part points to the next box in the chain. You can think of these boxes as nodes, and the entire chain as a linked list.
A linked list is a linear data structure used to store and organize data. Elements are not stored at contiguous memory locations. It consists of a series of nodes, where each node contains two main components: the data and a reference (or pointer) to the next node in the sequence.
The first node is called the head of the linked list, and the last node usually points to a null value, indicating the end of the list.
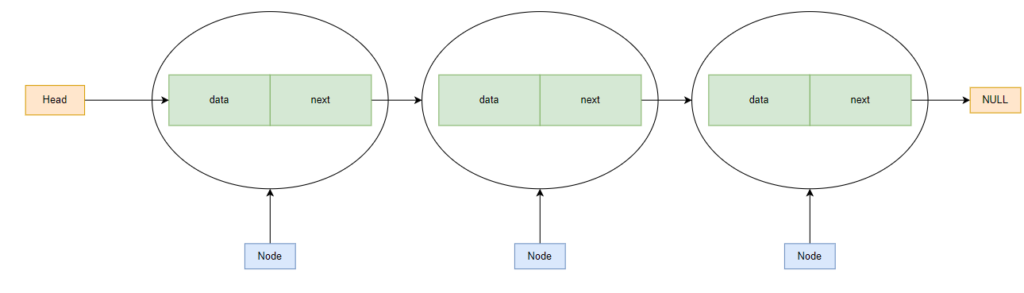
The key advantage of a linked list is that it allows dynamic memory allocation. Unlike arrays, which have a fixed size, a linked list can grow or shrink as needed by adding or removing nodes.
To access elements in a linked list, you start from the head node and follow the pointers to traverse through the list until you reach the desired node.
Here’s a simple example: Let’s say we have a linked list representing a sequence of numbers: 5 -> 9 -> 2 -> 7. In this case, the head node is 5, and it points to the next node containing 9. The second node points to 2, and the last node points to 7. To access the second element, you start from the head and follow the pointer to get to 9.
Linked lists are commonly used in programming because they provide flexibility in managing and manipulating data. They are used to implement various data structures like stacks, queues, and hash tables, and are particularly useful when you need to frequently insert or delete elements in a collection.
Representation of Singly Linked List
var head; // head of the list
/* Linked list Node*/
class Node
{
// Constructor to create a new node
// Next is by default initialized
// as null
constructor(val) {
this.data = val;
this.next = null;
}
}
console.log("hello")
Types of Linked List
- Singly Linked List
- Doubly Linked List
- Circular Linked List
- Doubly Circular Linked List
Basic Linked List Operations
- Insertion: Adding a new node to the linked list.
- At the beginning: Insert a node at the beginning of the linked list.
- At the end: Insert a node at the end of the linked list.
- After a specific node: Insert a node after a given node in the linked list.
- Deletion: Removing a node from the linked list.
- At the beginning: Delete the first node of the linked list.
- At the end: Delete the last node of the linked list.
- Specific node: Delete a node with a given value from the linked list.
- Traversal: Visiting each node in the linked list to perform some operation.
- Print: Traverse the linked list and print the value of each node.
- Search: Traverse the linked list to search for a specific value or condition.
- Length: Determining the number of nodes in the linked list.
- Access: Accessing a specific node or value in the linked list.
- By index: Accessing a node at a specific index in the linked list.
- Concatenation: Combining two linked lists into one.
- Reversal: Reversing the order of nodes in the linked list.
These are some of the basic operations commonly performed on a linked list. Depending on the requirements, more advanced operations or additional functionalities can be implemented.
Conclusion
Linked lists provide a flexible and efficient way to store and manipulate data. With their dynamic nature and efficient insertion and deletion operations, linked lists are an invaluable data structure in various programming scenarios. Understanding their anatomy, advantages, and common operations equips developers with powerful tools for efficient data organization and manipulation.
So, the next time you encounter a problem that involves frequent insertion and deletion operations or requires flexibility in managing data, consider harnessing the power of linked lists. Explore further, practice implementing different types of linked lists, and deepen your understanding of this fundamental data structure.