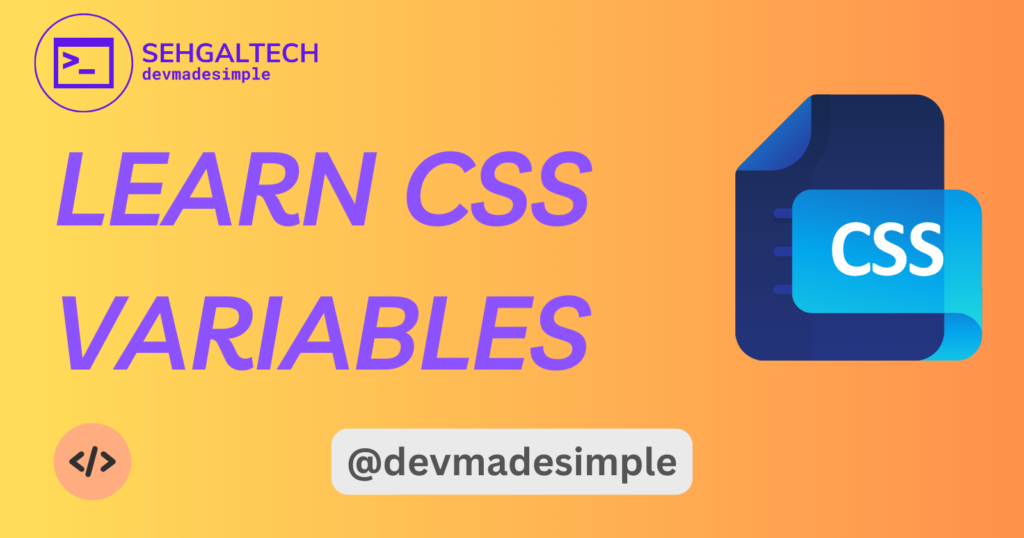
Creating visually appealing and consistent designs across a website is a challenge every web developer faces. Cascading Style Sheets (CSS) is a powerful language that controls the presentation of a web page. While traditional CSS allows for the definition of static values for properties like colors, sizes, and fonts, CSS Variables, also known as Custom Properties, bring a new level of flexibility and maintainability to web development. In this blog, we’ll dive deep into mastering CSS theming with variables and even build a dynamic theme switcher to witness the magic in action.
Understanding CSS Variables:
Introduction to CSS Variables:
CSS Variables allow you to store and reuse values throughout your stylesheet. They are defined using the --variable-name
syntax and are typically declared within the :root
selector to make them globally available. CSS variables redefine how we manage styles by allowing us to declare reusable values. They are processed by the browser at runtime, offering a dynamic approach to theming. Here’s a simple example:
:root {
--primary-color: #3498db;
--secondary-color: #2ecc71;
}
body {
background-color: var(--primary-color);
color: var(--secondary-color);
}
In this example, we’ve defined three variables: --primary-color
and --secondary-color
. These variables are then used to set the background-color
and color
properties of the body
element.
Dynamic Theming with Variables:
CSS variables shine when it comes to dynamic theming. Let’s explore how to change variable values at runtime, enabling dynamic themes like dark mode.
button {
background-color: var(--primary-color);
color: white;
}
body.dark-mode {
--primary-color: #2c3e50;
}
Implementing CSS Theming:
Creating a Theming System:
Organize CSS variables for colors, typography, spacing, and more to build a coherent theming system. This enhances code maintainability and flexibility.
:root {
--primary-color: #3498db;
--font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
--spacing-unit: 8px;
}
/* ... */
Responsive Theming:
Make your themes responsive using CSS media queries and variables. Adjust variable values based on viewport dimensions to ensure a consistent user experience across devices.
@media screen and (max-width: 600px) {
:root {
--font-size: 14px;
}
}
Building a Dynamic Theme Switcher:
HTML Structure:
Create a simple HTML structure for the theme switcher.
<div class="theme-switcher">
<label>
<input type="checkbox" id="themeToggle" />
Dark Mode
</label>
</div>
CSS Styles
Style the theme switcher to make it visually appealing.
.theme-switcher {
position: fixed;
top: 20px;
right: 20px;
}
/* ... */
:root {
--primary-color: #3498db;
--secondary-color: #2ecc71;
}
body {
background-color: var(--primary-color);
color: var(--secondary-color);
}
button {
background-color: var(--primary-color);
color: white;
}
.dark-mode {
--primary-color: #2c3e50;
}
JavaScript Logic:
Implement JavaScript to toggle the dark-mode
class on the body
element.
const themeToggle = document.getElementById('themeToggle');
themeToggle.addEventListener('change', () => {
document.body.classList.toggle('dark-mode');
});
Code Output


Advantages of CSS Variables
1. Reusability:
CSS Variables promote reusability by allowing you to define values once and use them across your entire stylesheet. This reduces redundancy and makes it easier to maintain a consistent design.
2. Easy Maintenance:
When you need to update a color or any other property, you can do it in one place – the variable declaration. The changes will automatically cascade throughout your styles, making maintenance a breeze.
3. Dynamic Theming:
CSS Variables enable the creation of dynamic themes. By changing variable values using JavaScript, you can implement features like light/dark mode easily.
Conclusion:
CSS Variables provide a powerful mechanism for creating flexible and maintainable stylesheets. By promoting reusability, easy maintenance, and dynamic theming, they enhance the efficiency of web development. Incorporating CSS Variables into your projects can streamline your workflow and contribute to a more consistent and adaptable design. As the web development landscape continues to evolve, mastering CSS Variables is a valuable skill for any front-end developer. Embrace the power of CSS variables, build captivating interfaces, and offer users a delightful experience with personalized themes. Happy theming! 🎨