Hey, welcome to my blog! Today I’m going to write about the component in React Native, which is one of the most basic and versatile components you can use in your app. If you’re new to React Native, you might be wondering what a is and how to use it. Well, don’t worry, I’m here to help you out!
A <View> is a container that supports layout with flexbox, style, some touch handling, and accessibility controls. It maps directly to the native view equivalent on whatever platform you are using, such as UIView on iOS, android.view on Android, or div on the web. You can think of it as a blank canvas that you can fill with other components, such as text, images, buttons, etc.
To use a <View>, you need to import it from the react-native module:
import { View } from 'react-native';
Then you can use it in your render function like this:
<View style={styles.container}>
// other components go here
</View>
The style prop is optional, but it allows you to customize the appearance and layout of your <View>. You can use any of the [style properties] that React Native supports, such as backgroundColor, borderColor, borderWidth, borderRadius, margin, padding, flex, flexDirection, alignItems, justifyContent, etc. For example, if you want to create a red square with a black border and some padding inside, you can do something like this:
<View
style={{
width: 100,
height: 100,
backgroundColor: 'red',
borderColor: 'black',
borderWidth: 2,
padding: 10,
}}>
// other components go here
</View>
You can also use the [StyleSheet] API to create and reuse styles across your app. For example, you can define a styles object like this:
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
},
square: {
width: 100,
height: 100,
backgroundColor: 'red',
borderColor: 'black',
borderWidth: 2,
padding: 10,
},
});
And then use it in your <View> like this:
<View style={styles.container}>
<View style={styles.square}>// other components go here</View>
</View>
One of the cool things about <View> is that it supports nesting. That means you can put a <View> inside another <View>, and so on. This allows you to create complex layouts with ease. For example, if you want to create a row of three squares with different colors and sizes, you can do something like this:
<View style={styles.container}>
<View style={{flexDirection: 'row'}}>
<View style={{width: 50, height: 50, backgroundColor: 'blue'}} />
<View style={{width: 75, height: 75, backgroundColor: 'green'}} />
<View style={{width: 100, height: 100, backgroundColor: 'yellow'}} />
</View>
</View>
Full Code
import React from 'react';
import { View, StyleSheet} from 'react-native';
function App() {
return (
<View style={styles.container}>
<View style={{flexDirection: 'row'}}>
<View style={{width: 50, height: 50, backgroundColor: 'blue'}} />
<View style={{width: 75, height: 75, backgroundColor: 'green'}} />
<View style={{width: 100, height: 100, backgroundColor: 'yellow'}} />
</View>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
alignItems: 'center',
justifyContent: 'center',
},
});
export default App;
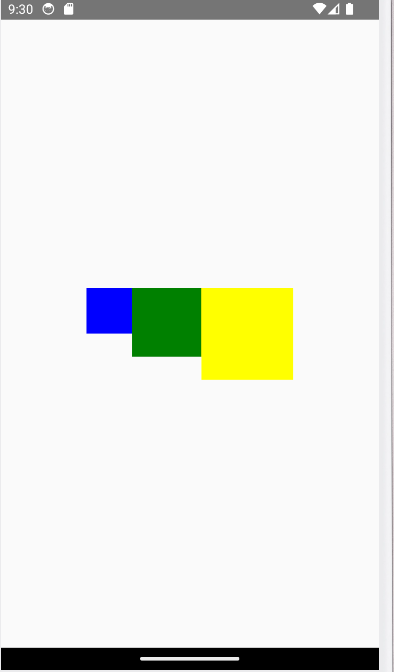
As you can see, the <View> component is very powerful and flexible. You can use it to create any kind of layout you want in your app. Of course, there are many other components that React Native provides, such as [Text], [Image], [Button], [ScrollView], [FlatList], etc. But they all ultimately rely on the <View> component as their base. So mastering the <View> component is essential for any React Native developer.
I hope this blog post was helpful and informative for you. If you have any questions or comments, feel free to leave them below. And if you liked this post, please share it with your friends and colleagues. Thanks for reading!