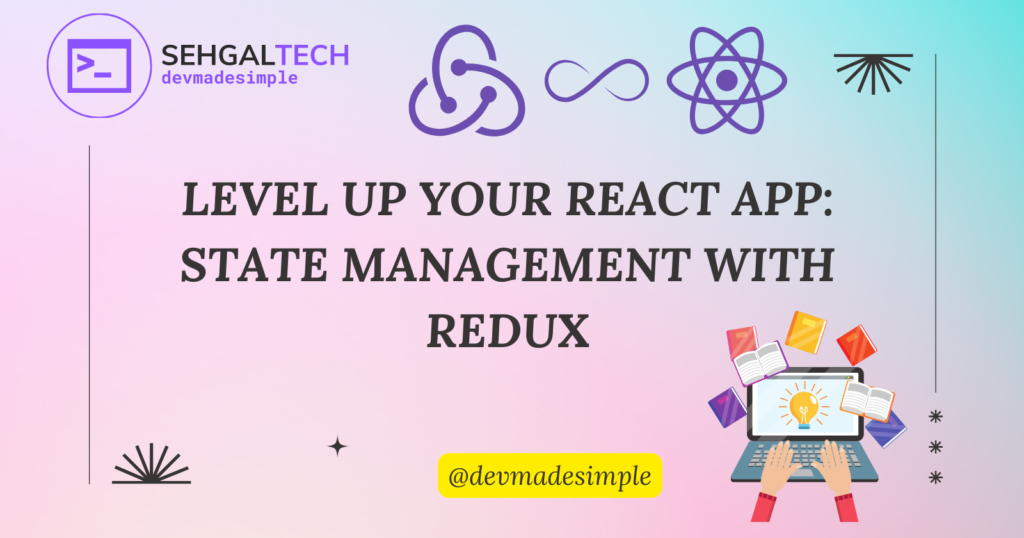
Welcome back to the React 101 series! In our journey through React, we’ve covered the fundamentals of components, props, and styling. Now, it’s time to tackle a crucial aspect of building robust applications: state management. In this post, we’ll explore the use of Redux, a popular state management library, to handle complex data flows in your React applications.
The Need for State Management
As React applications grow in complexity, managing and sharing state between components becomes challenging. Passing props down through multiple layers can lead to “prop drilling,” making your code harder to maintain. This is where state management libraries like Redux come to the rescue.
Introducing Redux
Redux is a predictable state container for JavaScript applications. It provides a centralized store to manage the state of your application, making it easier to organize, update, and access data across different components.
Redux follows a unidirectional data flow architecture. Here’s the core principle:
- Actions: Events that signal state changes. They carry information about the change (e.g., adding an item to the cart).
- Reducer: A pure function that takes the current state and an action, and returns the new state based on the logic defined within the reducer.
- Store: Holds the single source of truth for your application state. It receives actions and dispatches them to the reducer to update the state.
1. Store:
The store is the single source of truth in a Redux application. It holds the entire state tree of your application. To create a store, you need a reducer function.
// store.js
import { createStore } from 'redux';
import rootReducer from './reducers';
const store = createStore(rootReducer);
export default store;
2. Reducers:
Reducers are pure functions responsible for specifying how the application’s state changes in response to actions. Each reducer handles a specific slice of the application state.
// reducers.js
const initialState = {
counter: 0,
};
const counterReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { ...state, counter: state.counter + 1 };
case 'DECREMENT':
return { ...state, counter: state.counter - 1 };
default:
return state;
}
};
export default counterReducer;
3. Actions:
Actions are payloads of information that send data from your application to the Redux store. They are the only source of information for the store.
// actions.js
export const increment = () => {
return {
type: 'INCREMENT',
};
};
export const decrement = () => {
return {
type: 'DECREMENT',
};
};
4. Connecting Components:
The connect
function from the react-redux
library connects your React components to the Redux store, allowing them to access state and dispatch actions.
Connected components receive parts of the application state as props, allowing them to render based on the current state.
// CounterComponent.js
import React from 'react';
import { connect } from 'react-redux';
import { increment, decrement } from './actions';
const CounterComponent = ({ counter, increment, decrement }) => {
return (
<div>
<p>Counter: {counter}</p>
<button onClick={increment}>Increment</button>
<button onClick={decrement}>Decrement</button>
</div>
);
};
const mapStateToProps = (state) => {
return {
counter: state.counter,
};
};
export default connect(mapStateToProps, { increment, decrement })(CounterComponent);
Benefits of Using Redux in React
- Predictable State Updates: Redux enforces consistent state updates, simplifying debugging and reasoning about your application’s behavior.
- Improved Maintainability: Centralized state management reduces code duplication and makes it easier to track changes.
- Scalability: Redux scales well with complex applications with many components and intricate data relationships.
Handling Complex Data Flows
As applications become more complex, managing state for various features and components can be challenging. Redux middleware, such as Thunk or Saga, can be employed to handle asynchronous operations, side effects, and complex data flows.
1. Redux Thunk:
Thunk middleware enables the use of asynchronous actions in Redux. It allows you to dispatch functions, which can be useful for handling API calls and other asynchronous tasks.
// actions.js
import axios from 'axios';
export const fetchData = () => {
return async (dispatch) => {
try {
const response = await axios.get('https://api.example.com/data');
dispatch({ type: 'FETCH_DATA_SUCCESS', payload: response.data });
} catch (error) {
dispatch({ type: 'FETCH_DATA_FAILURE', payload: error.message });
}
};
};
2. Redux DevTools:
The Redux DevTools extension is a powerful tool for debugging and inspecting the state and actions of your Redux store. It provides a visual representation of your application’s state changes.
Conclusion
Redux is a powerful state management library that can greatly simplify handling complex data flows in your React applications. By centralizing the state in a store, managing actions, and employing middleware, you can maintain a clear and organized structure for your code. Stay tuned for more insights into mastering React development!