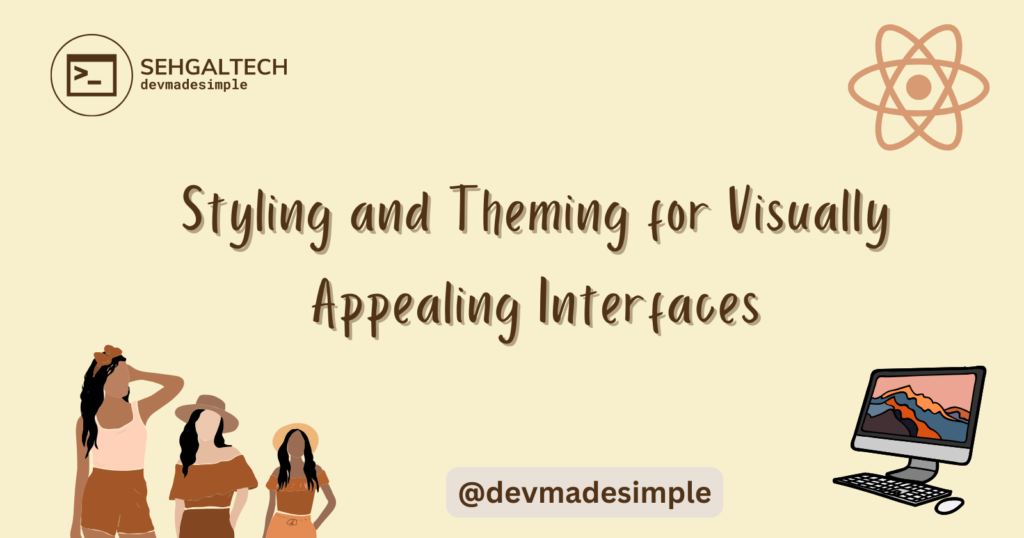
Welcome back to the React 101 series! In this blog post, we’ll explore the world of styling and theming to create visually appealing interfaces. We’ll discuss the importance of good design, popular styling approaches in React, and provide practical code examples.
Why Styling Matters
The user interface (UI) is the first thing users notice when interacting with your application. A well-designed and visually appealing UI not only enhances user experience but also reflects positively on the overall quality of your application. Good styling helps guide users, improves readability, and creates a cohesive look and feel.
Popular Styling Approaches in React
- Inline Styles:
- Styles are directly added within the JSX using the
style
attribute. - Pros: Simple for small changes.
- Cons: Can become messy and hard to maintain for larger projects.
function MyButton() {
return <button style={{ backgroundColor: 'blue', color: 'white' }}>Click Me</button>;
}
2. CSS Modules:
- Styles are defined in separate CSS files with unique class names scoped locally to the component.
- Pros: Improves code organization, prevents style conflicts across components.
- Cons: Requires setting up module loaders and can feel verbose for simple styles.
/* MyButton.module.css */
.button {
background-color: blue;
color: white;
}
// MyButton.jsx
import styles from './MyButton.module.css';
function MyButton() {
return <button className={styles.button}>Click Me</button>;
}
3. CSS-in-JS Libraries:
- Styled Components is a library that allows you to write CSS directly within your JavaScript files. It uses tagged template literals to create components with styled elements.
- Pros: Improves readability, promotes component-specific styles, and offers features like theming.
- Cons: Adds another dependency and has a slight learning curve.
import styled from 'styled-components';
const Button = styled.button`
background-color: blue;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
`;
function MyButton() {
return <Button>Click Me</Button>;
}
4. UI Component Libraries:
- Libraries like Material UI and Ant Design offer pre-built, styled components.
- Pros: Saves development time, provides consistent design patterns, and often include theming capabilities.
- Cons: Introduces an external dependency and might not perfectly match your specific design needs.
Theming in React
Theming adds another layer to styling by providing a consistent set of design choices across an application. React allows you to implement theming in various ways, such as using context or external libraries.
- Context API: The Context API in React allows you to create a theme context that can be accessed by all components within the application. This context provides a centralized place to store and update theme-related information.
// ThemeContext.js
import { createContext, useContext, useState } from 'react';
const ThemeContext = createContext();
export const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
export const useTheme = () => {
return useContext(ThemeContext);
};
Usage:
// MyThemedComponent.js
import React from 'react';
import { useTheme } from './ThemeContext';
const MyThemedComponent = () => {
const { theme, toggleTheme } = useTheme();
return (
<div style={{ background: theme === 'light' ? '#fff' : '#333', color: theme === 'light' ? '#333' : '#fff' }}>
Hello, React! Theme: {theme}
<button onClick={toggleTheme}>Toggle Theme</button>
</div>
);
};
2. External Libraries: There are several external libraries, such as styled-components
, that offer built-in support for theming. These libraries often provide a ThemeProvider component and make it easy to define and switch between themes.
// ThemeProvider.js
import { createGlobalStyle, ThemeProvider as StyledThemeProvider } from 'styled-components';
const GlobalStyle = createGlobalStyle`
body {
background-color: ${(props) => props.theme.background};
color: ${(props) => props.theme.text};
}
`;
const theme = {
light: {
background: '#fff',
text: '#333',
},
dark: {
background: '#333',
text: '#fff',
},
};
export const ThemeProvider = ({ children }) => {
return (
<StyledThemeProvider theme={theme.light}>
<GlobalStyle />
{children}
</StyledThemeProvider>
);
};
Usage:
// MyThemedComponent.js
import React from 'react';
import { ThemeProvider } from './ThemeProvider';
import styled from 'styled-components';
const ThemedDiv = styled.div`
padding: 20px;
font-size: 18px;
`;
const MyThemedComponent = () => {
return (
<ThemeProvider>
<ThemedDiv>Hello, React! Themed.</ThemedDiv>
</ThemeProvider>
);
};
Popular theming libraries for React include:
- Styled Components: Provides built-in theming support.
- Material UI: Offers a built-in theme object for customization.
- Theme UI: A dedicated library for creating and managing themes
Conclusion
Styling and theming play a crucial role in creating visually appealing and user-friendly React applications. Whether you choose inline styles, CSS Modules, UI libraries or styled components, make sure to select an approach that aligns with your project requirements. Additionally, theming adds an extra layer of consistency to your design, ensuring a seamless and professional look across your application.