Introduction:
In the ever-evolving world of web development and security, choosing the right authentication method is a critical decision for developers. Two popular approaches, session-based authentication and token-based authentication, each have their merits and drawbacks. In this blog post, we will delve into the characteristics of both methods to help you make an informed decision based on your project requirements.
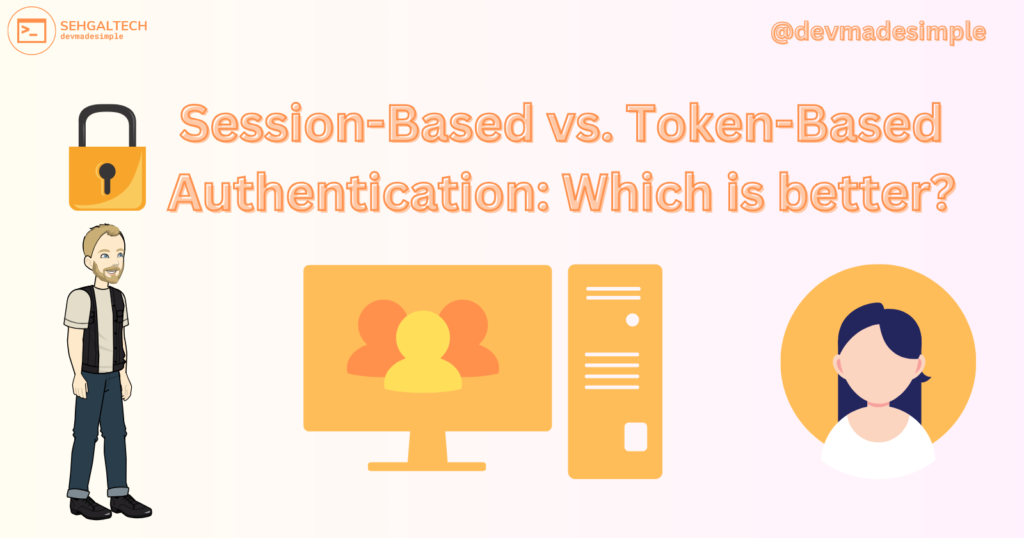
Session-Based Authentication:
Session-based authentication has been a traditional method widely used on the web. In this approach, a server creates a unique session for each user upon successful login. The server then stores this session information, often in the form of a session ID, on the server side. The session ID is then sent to the client, usually as a cookie, which the client includes in subsequent requests.
Pros of Session-Based Authentication:
- Security: Session-based authentication can be considered more secure since the session data is stored on the server, reducing the risk of exposure to client-side attacks.
- Simplicity: Implementing session-based authentication is often simpler and requires less boilerplate code. Most web frameworks provide built-in support for session management.
- Automatic Expiry: Sessions can be configured to expire after a certain period of inactivity, reducing the risk of unauthorized access.
Cons of Session-Based Authentication:
- Scalability: As the server needs to store session information for each user, this can lead to scalability challenges, especially in large-scale applications.
- Stateful: Session-based authentication is inherently stateful, meaning the server needs to keep track of the session state for each user. This can complicate the architecture and increase server load.
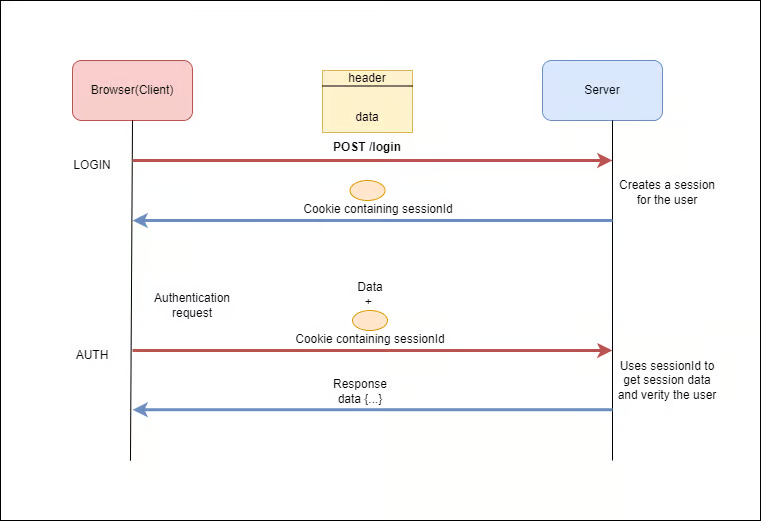
Example
Here’s an example of a Node.js application using Express.js to implement session authentication.
Implementation
const express = require('express');
const session = require('express-session');
const app = express();
// Middleware setup
app.use(session({
secret: 'your_secret_key',
resave: false,
saveUninitialized: false,
cookie: {
httpOnly: true, // Set the cookie as HTTP-only, Optional
maxAge: 60*30 // In secs, Optional
}
}));
Login Route
app.post('/login', (req, res) => {
const { username, password } = req.body;
const user = users.find(u => u.username === username && u.password === password);
if (user) {
req.session.userId = user.id; // Store user ID in session
res.send('Login successful');
} else {
res.status(401).send('Invalid credentials');
}
});
Protected Route
app.get('/home', (req, res) => {
if (req.session.userId) {
// User is authenticated
res.send(`Welcome to the Home page, User ${req.session.userId}!`);
} else {
// User is not authenticated
res.status(401).send('Unauthorized');
}
});
Logout Route
app.get('/logout', (req, res) => {
req.session.destroy(err => {
if (err) {
res.status(500).send('Error logging out');
} else {
res.redirect('/'); // Redirect to the home page after logout
}
});
});
Token-Based Authentication:
Token-based authentication has gained popularity with the rise of modern web development and the prevalence of Single Page Applications (SPAs) and mobile apps. In this approach, upon successful login, the server generates a token (usually a JSON Web Token or JWT) containing user information and sends it to the client. The client includes this token in the headers of subsequent requests for authentication.
Pros of Token-Based Authentication:
- Stateless: Token-based authentication is stateless, meaning the server does not need to store any session information. This makes it more scalable and easier to implement in distributed systems.
- Cross-Domain Authorization: Tokens allow for seamless authentication across different domains, making it ideal for microservices and decentralized architectures.
- Flexibility: Tokens can store additional user information, making them versatile for customizing user roles and permissions.
Cons of Token-Based Authentication:
- Security Risks: If not implemented correctly, tokens can pose security risks, such as token leakage or insufficient validation.
- Token Management: Developers need to manage token expiration and refresh mechanisms to ensure a secure authentication process.
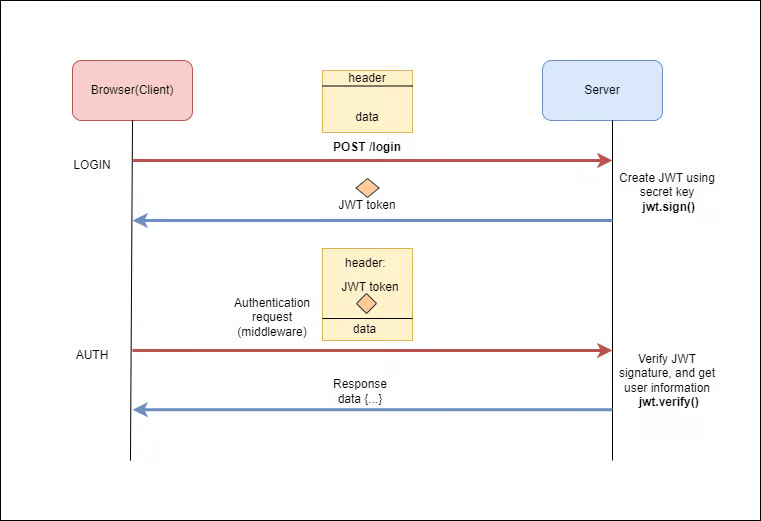
Example
Login
app.post('/login', (req, res) => {
const { username, password } = req.body;
const user = users.find(u => u.username === username && u.password === password);
jwt.sign({ user }, secretKey, { expiresIn: '1h' }, (err, token) => {
if (err) {
res.status(500).send('Error generating token');
} else {
res.json({ token });
}
});
});
Protected route
We are using veriyToken()
function as middleware for every route that needs verification. The request passes through the veriyToken()
and only if the next()
function is called, it passes on to this route and implements the code.
app.get('/dashboard', verifyToken, (req, res) => {
res.send('Welcome to the Home page');
});
// Verify token middleware
function verifyToken(req, res, next) {
const token = req.headers['authorization'];
if (typeof token !== 'undefined') {
jwt.verify(token.split(' ')[1], secretKey, (err, decoded) => {
if (err) {
res.status(403).send('Invalid token');
} else {
req.user = decoded.user;
next();
}
});
} else {
res.status(401).send('Unauthorized');
}
}
Key differences
- Storage Location: Sessions are stored on the server, while tokens (JWTs) are stored on the client side.
- Stateful vs Stateless: Sessions are stateful, while tokens are stateless, allowing for better scalability in distributed systems.
- Expiry Handling: Session expiry is managed by the server, whereas token expiry is handled by the token itself.
- Security Measures: JWTs often include digital signatures and support for encryption, enhancing security compared to typical session mechanisms that use cookies, and can be vulnerable to CSRF attacks if not properly protected.
- Usage Flexibility: Tokens (JWTs) offer more flexibility in carrying additional information beyond authentication, useful for authorization and custom data transmission.
Conclusion:
The choice between session-based and token-based authentication depends on the specific requirements and constraints of your project. Session-based authentication may be preferable for smaller applications with simpler authentication needs, while token-based authentication is often favored for larger, more complex systems. Ultimately, understanding the strengths and weaknesses of each approach will empower you to make an informed decision that aligns with your project goals and security considerations.
I hope you liked this posts and if you did donβt forget to subscribe to our feed! Which backend language do you use for your projects? π€
Comment them down below π